How to Read Whole Text File in Python
To read a text file in Python:
- Open the file.
- Read the lines from the file.
- Close the file.
For example, let'due south read a text file chosen example.txt from the same binder of the lawmaking file:
with open('example.txt') equally file: lines = file.readlines()
Now the lines variable stores all the lines from case.txt as a list of strings.
This is the quick answer. To learn more than details about reading files in Python, please read along.
Reading Text Files in Python
Reading a text file into your Python program follows this process:
- Open the file with the congenital-in open up() function by specifying the path of the file into the call.
- Read the text from the file using i of these methods: read(), readline(), readlines().
- Shut the file using the shut() method. Y'all tin can let Python handle closing the file automatically by opening the file using the with statement.
i. How to Utilise the open() Function in Python
The basic syntax for calling the open() function is:
open(path_to_a_file, mode)
Where:
- path_to_a_file is the path to the file you want to open. For instance Desktop/Python/example.txt. If your python plan file is in the same binder equally the text file, the path is just the name of the file.
- manner specifies in which country you want to open the file. There are multiple options. But as you're interested in reading a file, you only need the fashion 'r'.
The open() function returns an iterable file object with which you lot can hands read the contents of the file.
For example, if yous have a file called case.txt in the aforementioned folder as your code file, you lot can open it by:
file = open("instance.txt", "r")
At present the file is opened, merely not used in whatsoever useful way yet.
Side by side, permit's take a wait at how to actually read the opened file in Python.
ii. File Reading Methods in Python
To read an opened file, let'due south focus on the three different text reading methods: read(), readline(), and readlines():
- read() reads all the text from a file into a unmarried string and returns the string.
- readline() reads the file line past line and returns each line as a separate string.
- readlines() reads the file line past line and returns the whole file as a list of strings, where each string is a line from the file.
Afterward you are going to see examples of each of these methods.
For instance, allow's read the contents of a file chosen "example.txt" to a variable as a string:
file = open up("instance.txt") contents = file.read()
Now the contents variable has whatever is within the file every bit a single long string.
3. Always Close the File in Python
In Python, an opened file remains open as long as you don't close it. So make certain to close the file afterwards using it. This tin can exist done with the close() method. This is important because the program can crash or the file can corrupt if left hanging open.
file.close()
You tin can also permit Python accept intendance of closing the file by using the with statement when dealing with files. In this case, you don't demand the close() method at all.
The with statement structure looks like this:
with open(path_to_file) as file: #read the file here
Using the with statement is so convenient and conventional, that nosotros are going to stick with information technology for the rest of the guide.
Now yous empathize the basics of reading files in Python. Next, let'south have a look at reading files in activeness using the different reading functions.
Using the File Reading Methods in Python
To echo the post-obit examples, create a folder that has the following 2 files:
- A reader.py file for reading text files.
- An instance.txt file from where your program reads the text.
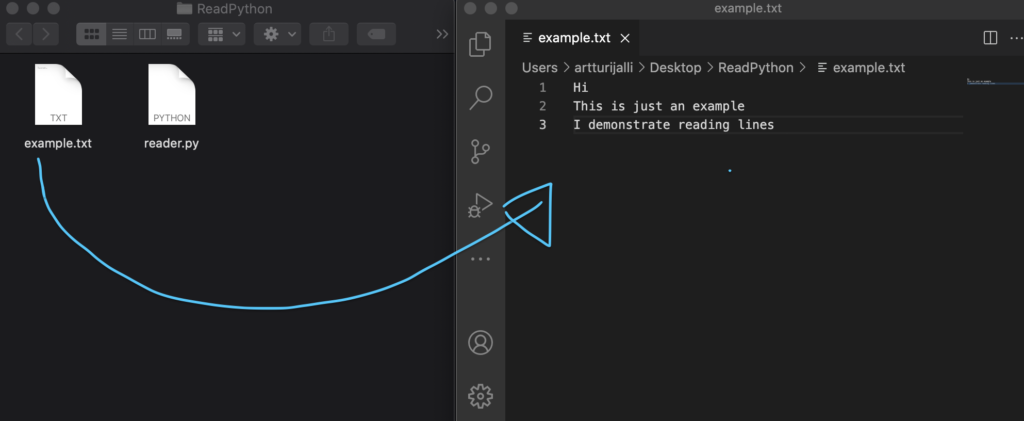
Too, write some text on multiple lines into the example.txt file.
The read() method in Python
To read a file to a unmarried string, utilize the read() method. As discussed above, this reads whatever is in the file equally a single long cord into your program.
For example, let's read and print out the contents of the example.txt file:
with open("example.txt") as file: contents = file.read() print(contents)
Running this piece of lawmaking displays the contents of example.txt in the console:
Hullo This is only an example I demonstrate reading lines
The readline() Method in Python
To read a file one line at a fourth dimension, use the readline() method. This reads the current line in the opened file and moves the line pointer to the next line. To read the whole file, utilize a while loop to read each line and movement the file pointer until the finish of the file is reached.
For example:
with open('example.txt') every bit file: next_line = file.readline() while next_line: print(next_line) next_line = file.readline()
As a result, this program prints out the lines one by one equally the while loop proceeds:
How-do-you-do This is just an example I'm demonstrate reading lines
The readlines() Method in Python
To read all the lines of a file into a list of strings, use the readlines() method.
When you have read the lines, y'all can loop through the list of lines and impress them out for instance:
with open up('example.txt') as file: lines = file.readlines() line_num = 0 for line in lines: line_num += 1 print(f"line {line_num}: {line}")
This displays each line in the console:
line 1: Hi line 2: This is just an instance line three: I'chiliad demonstrate reading lines
Utilize a For Loop to Read an Opened File
You just learned about three different methods you lot tin apply to read a file into your Python programme.
It is good to realize that the open up() function returns an iterable object. This means that yous tin loop through an opened file merely like you lot would loop through a list in Python.
For instance:
with open('example.txt') as file: for line in file: print(line)
Output:
Hi This is but an example I'm demonstrate reading lines
As you tin see, you did non need to use any of the congenital-in file reading methods. Instead, you used a for loop to run through the file line by line.
Conclusion
Today you learned how to read a text file into your Python program.
To recap, reading files follows these three steps:
- Use theopen() function with the'r' style to open a text file.
- Use ane of these three methods:read(),readline(), orreadlines() to read the file.
- Close the file after reading it using theclose() method or allow Python do it automatically by using thewith statement.
Conventionally, yous can also employ the with statement to reading a file. This automatically closes the file for you lot and then yous do non demand to worry about the third pace.
Thanks for reading. Happy coding!
Further Reading
Python—How to Write to a File
10 Useful Python Snippets to Lawmaking Like a Pro
Source: https://www.codingem.com/read-textfile-into-python-program/
ارسال یک نظر for "How to Read Whole Text File in Python"